45 how to do label encoding in python for multiple columns
How to do Label Encoding across multiple columns - Kaggle 3. Hi @samacker77k ! There are multiple ways to do it. I usually follow below method: Let me know if you need more info around this. P.S: I'm sure we are not confused between Label Encoding and One Hot. If we are, below code should do for One Hot encoding: pd.get_dummies (df,drop_first=True) One hot Encoding with multiple labels in Python? - DeZyre Step 1 - Import the library Step 2 - Setting up the Data Step 3 - Using MultiLabelBinarizer and Printing Output Step 1 - Import the library from sklearn.preprocessing import MultiLabelBinarizer We have only imported MultiLabelBinarizer which is reqired to do so. Step 2 - Setting up the Data
› label-encoding-in-pythonWhat is Label Encoding in Python | Great Learning Dec 16, 2021 · Label Encoding using Python. Before we proceed with label encoding in Python, let us import important data science libraries such as pandas and NumPy. Then, with the help of panda, we will read the Covid19_India data file which is in CSV format and check if the data file is loaded properly. With the help of info().
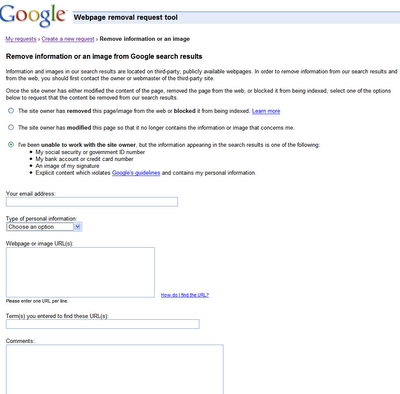
How to do label encoding in python for multiple columns
How to perform one hot encoding on multiple categorical columns You can do dummy encoding using Pandas in order to get one-hot encoding as shown below: import pandas as pd # Multiple categorical columns categorical_cols = ['a', 'b', 'c', 'd'] pd.get_dummies(data, columns=categorical_cols) If you want to do one-hot encoding using sklearn library, you can get it done as shown below: Label Encoding in Python - Machine Learning - PyShark Label Encoding in Python In this part we will cover a few different ways of how to do label encoding in Python. Two of the most popular approaches: LabelEncoder () from scikit-learn library pandas.factorize () from pandas library Once the libraries are downloaded and installed, we can proceed with Python code implementation. Label Encoding of datasets in Python - GeeksforGeeks We apply Label Encoding on iris dataset on the target column which is Species. It contains three species Iris-setosa, Iris-versicolor, Iris-virginica . Python3 import numpy as np import pandas as pd df = pd.read_csv ('../../data/Iris.csv') df ['species'].unique () Output: array ( ['Iris-setosa', 'Iris-versicolor', 'Iris-virginica'], dtype=object)
How to do label encoding in python for multiple columns. Encoding Categorical Values, Python- Scikit-Learn - Medium In Data science, we work with datasets which has multiple labels in one or more columns. They can be in numerical or text format of any encoding. This will be ideal and understandable by humans. Multiple Linear Regression using Python - Analytics Vidhya 11.03.2022 · Perform One-Hot Encoding. Change columns using Column Transformer. Split the dataset into train set and test set. Train the model . Predict the test Results. Evaluate the model. Plot the Results. Predicted Values. Introduction. In this article, we will be dealing with multi-linear regression, and we will take a dataset that contains information about 50 startups. Features include R&D Spend ... How to use label encoding through Python on multiple categorical data ... to find what kind of encoding should be used to handle such categorical data. If we consider hierarchical clustering algorithms, they use distances between objects/items to be clustered and form... Python | Character Encoding - GeeksforGeeks So, in such cases when the encoding is not known, such non-encoded text has to be detected and the be converted to a standard encoding. So, this step is important before processing the text further. Charade Installation : For performing the detection and conversion of encoding, charade - a Python library is required. This module can be simply ...
Label encoding across multiple columns in scikit-learn - python.engineering The classically Pythonic way, available in Python 2 and Python 3.0-3.4, is to do this as a two-step process: z = x.copy() z.update(y) # which returns None since it mutates z In both approaches, y will come second and its values will replace x "s values, thus b will point to 3 in our final result. Not yet on Python 3.5, but want a single expression Label encode multiple columns in a Parandas DataFrame - Stephen Allwright Label encode multiple columns in a Pandas DataFrame Oct 23, 2021 1 min read Pandas Label encode multiple columns Label encoding is a feature engineering method for categorical features, where a column with values ['egg','flour','bread'] would be turned in to [0,1,2] which is usable by a machine learning model. Python for NLP: Multi-label Text Classification with Keras 27.08.2019 · Multi-label text classification is one of the most common text classification problems. In this article, we studied two deep learning approaches for multi-label text classification. In the first approach we used a single dense output layer with multiple neurons where each neuron represented one label. Label Encoding in Python - A Quick Guide! - AskPython Python sklearn library provides us with a pre-defined function to carry out Label Encoding on the dataset. Syntax: from sklearn import preprocessing object = preprocessing.LabelEncoder () Here, we create an object of the LabelEncoder class and then utilize the object for applying label encoding on the data. 1. Label Encoding with sklearn
How to Encode Categorical Columns Using Python - Medium First, we will reformat columns with two distinct values. They are the ever_married and the residence_type column. For doing that, we can use the LabelEncoder object from scikit-learn to encode the columns. Now let's take the ever_married column. First, we will initialize the LabelEncoder object like this: What is Label Encoding in Python | Great Learning 16.12.2021 · Label Encoding using Python. Before we proceed with label encoding in Python, let us import important data science libraries such as pandas and NumPy. Then, with the help of panda, we will read the Covid19_India data file which is in CSV format and check if the data file is loaded properly. With the help of info(). We can notice that a state ... python - How to reverse Label Encoder from sklearn for multiple columns ... LabelEncoder () should only be used to encode the target. That's why you can't use it on multiple columns at the same time as any other transformers. The alternative is the OrdinalEncoder which does the same job as LabelEncoder but can be used on all categorical columns at the same time just like OneHotEncoder: LabelEncoder Example - Single & Multiple Columns - Data … 23.07.2020 · Here is the code which can be used to encode multiple columns. Let’s say we will like to encode multiple columns such as worke’, status, hsc_s, degree_t. The ask is can we encode multiple columns all at once? LabelEncoder is used in the code given …
Label Encoding on multiple columns - Kaggle You can use the below code on your data frame, it label encoding will be applied on all column from sklearn.preprocessing import LabelEncoder df = df.apply (LabelEncoder ().fit_transform) Harry Wang • 2 years ago • Options • Report • Reply keyboard_arrow_up 7 You can use df.apply () to apply le.fit_transform to multiple columns:
Label encoding across multiple columns in scikit-learn - Stack Overflow encoding_pipeline = Pipeline ( [ ('encoding',MultiColumnLabelEncoder (columns= ['fruit','color'])) # add more pipeline steps as needed ]) encoding_pipeline.fit_transform (fruit_data) Share Improve this answer answered May 15, 2015 at 19:27 PriceHardman 1,583 1 11 14 2 Just realized the data implies that an orange is colored green. Oops. ;)
How to reverse Label Encoder from sklearn for multiple columns? This is the code I use for more than one columns when applying LabelEncoder on a dataframe: 25. 1. class MultiColumnLabelEncoder: 2. def __init__(self,columns = None): 3. self.columns = columns # array of column names to encode. 4.
› python-pandas-tips-and-tricksMaster Python's pandas library with these 100 tricks Sep 05, 2019 · df.columns = df.columns.str.lower().str.rstrip()#Python #pandastricks — Kevin Markham (@justmarkham) June 25, 2019 Selecting rows and columns. 🐼🤹♂️ pandas trick: You can use f-strings (Python 3.6+) when selecting a Series from a DataFrame! See example 👇#Python #DataScience #pandas #pandastricks @python_tip pic.twitter.com ...
Label Encoding in Python - Javatpoint Explanation: In the above example, we have imported pandas and preprocessing modules of the scikit-learn library. We have then defined the data as a dictionary and printed a data frame for reference. Later on, we have used the fit_transform() method in order to add label encoder functionality pointed by the object to the data variable. We have printed the unique code with respect to the Gender ...
Label encoding across multiple columns in scikit-learn We will now understand with the help of an example that how we can do label encoding across multiple columns in sklearn. For this purpose, we will import preprocessing function from sklearn library which will use Labelencoder method in order to achieve label encoding. Let us understand with help of an example,
Master Python's pandas library with these 100 tricks 05.09.2019 · df.columns = df.columns.str.lower().str.rstrip()#Python #pandastricks — Kevin Markham (@justmarkham) June 25, 2019 Selecting rows and columns. 🐼🤹♂️ pandas trick: You can use f-strings (Python 3.6+) when selecting a Series from a DataFrame! See example 👇#Python #DataScience #pandas #pandastricks @python_tip pic.twitter.com ...
Guide to Encoding Categorical Values in Python - Practical Business Python Approach #2 - Label Encoding. Another approach to encoding categorical values is to use a technique called label encoding. Label encoding is simply converting each value in a column to a number. For example, the body_style column contains 5 different values. We could choose to encode it like this: convertible -> 0; hardtop -> 1; hatchback -> 2
pbpython.com › categorical-encodingGuide to Encoding Categorical Values in Python - Practical ... Approach #2 - Label Encoding. Another approach to encoding categorical values is to use a technique called label encoding. Label encoding is simply converting each value in a column to a number. For example, the body_style column contains 5 different values. We could choose to encode it like this: convertible -> 0; hardtop -> 1; hatchback -> 2
Post a Comment for "45 how to do label encoding in python for multiple columns"